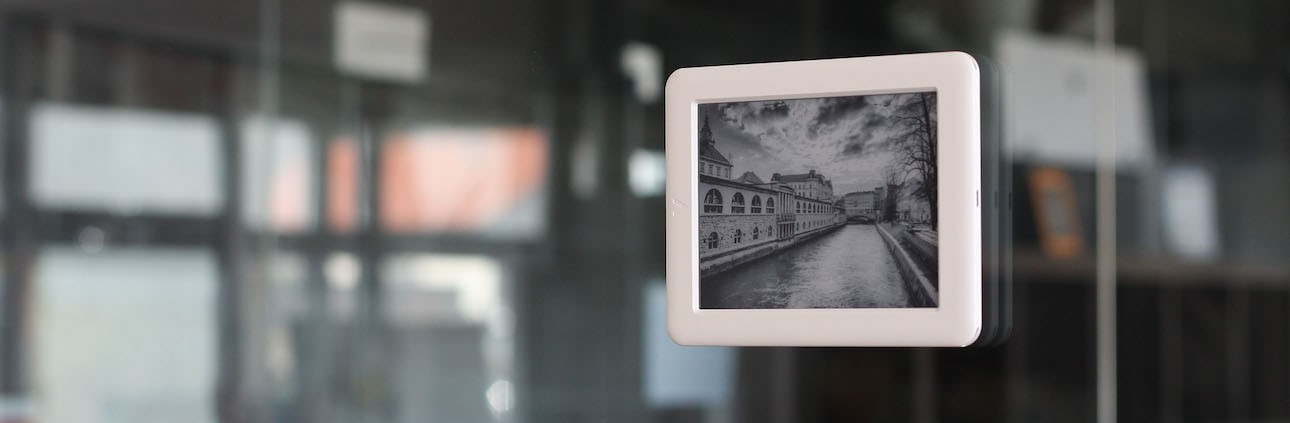
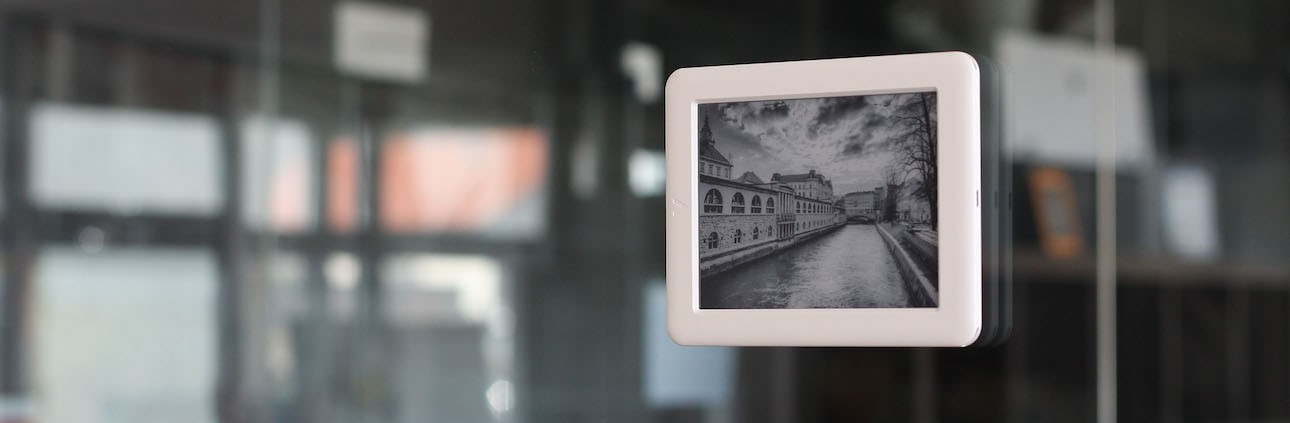
How Node.js helped us make a Twitter and Instagram powered e-paper picture frame
Visionect, 19 Mar 2014
A customer inquired how to build a Twitter app for our V Tablet devices. We picked Node.js, Engine.IO and IFTTT and got to create the world first Instagram powered e-paper photo frame in a day.
One of our customers asked if we have a suggestion on how to develop a Twitter application for our V Tablet devices (and any other browser client). It occurred to us that this might be interesting for anybody trying to tinker with Twitter, so we posted the how-to here.
Ready, set, go.
First things first – we need to access the Twitter feed somehow.
It took us a day to develop a Node.js app which allows you to whip out Instagram on our mobile phone and tag any new post with #ourhasthag to get it automatically pop-up on a e-paper picture frame.
We tried to integrate Twitter directly from the browser – run a query from the browser against their Javascript API, fetch data and display results. That couldn’t have been more than a couple lines of code for complete implementation.
Sadly, it turns out that Twitter discontinued their 1.0 API which supported unauthenticated API access. This means that unless you’re comfortable with storing your connection data publicly you require a server-client solution.
We were kind-of happy about this, as this looked like the perfect project where we can play with Node.js.
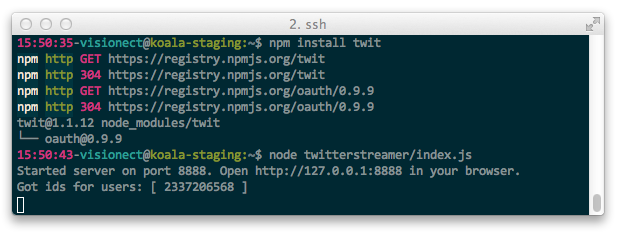
Using Node.js is super easy.
We installed the base system on OSX with MacBrew by simply running:
$ brew install node
Next step was to add the Twit client which is apparently the way people integrate Twitter into their Node.js apps. They have a great beginners’ guide which will get you operational in no-time.
Before we continue we need to sort out Twitter API access – you’re going to get you authentication details when you register a new app at Twitter. Copy the API authentication keys and configure Twit access details accordingly.
auth: { consumer_key: 'API key', consumer_secret: 'API secret', access_token: 'Access token', access_token_secret: 'Access token secret' },
Congratulations. You’re now able to access tweets in your Node.js app.
Pushing updates to clients.
We will be using a Visionect V Tablet device as our e-paper photo frame. Every device has its own WebKit session so pushing content is no different than building a simple browser app for reading tweets. We’ll use the Node.js server that we just built.
We could simply poll periodically the server for new tweets, but that’s not very in the spirit of times. Proper browsers (this includes Visionect Server, based on WebKit) support WebSockets, which can be used to push updates directly to the client.
Enter Engine.IO.
Engine.IO lets you use WebSockets in a simple, cross-browser way. Following the examples on their page it was fairly simple to create a simple listener:
//running client-side: var interval = null;a var initSocket = function() { var socket = new eio.Socket('ws://' + window.location.host); socket.on('open', function (data) { clearInterval(interval); interval = null; //parse every message you get socket.on('message', function (data) { data = JSON.parse(data); //update src for the image placeholder //NOTE: missing sanatization for production purposes $('img').attr('src', data); }); socket.on('close', function() { socket.off('open message close'); interval = setInterval(function() { initSocket(); }, 5000); }); }); } initSocket();
The server component tracks for tweets with a specific hash-tag. It then searches if there is an image URL attached to the tweet and sends that URL to the client. The client script takes the incoming data, which is an image URL and puts it into the placeholder.
Full code is available at our github account.
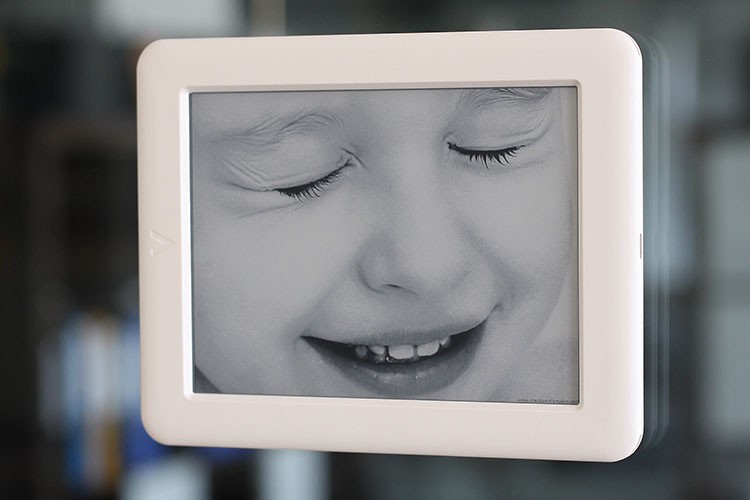
The client script takes the incoming data, which is an image URL and puts it into the
placeholder.
What about Instagram?
We figured we could do the same for Instagram as we did for Twitter. But being very good lazy programmers we instead browsed the web to find a service which would connect Instagram to Twitter. It turns out there is a bunch of app-to-app solutions and most popular seem to be IFTTT (if-this-then-that) and Zapier.
We opted for the former, created an account and used an existing recipe for posting Instagram pictures as native Twitter pictures.
Voila. 10 minutes later we were pushing image updates to our Twitter clients.
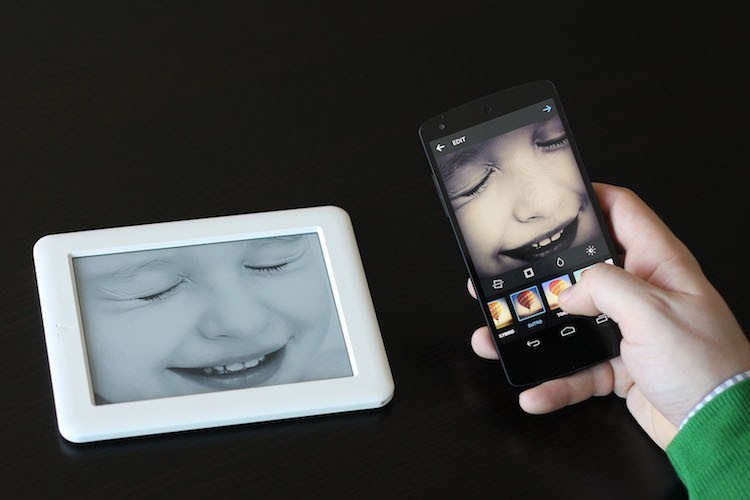
Posting Instagram pictures as native Twitter pictures.
Everything came together like a charm.
It took us a day to develop a Node.js app which allows you to whip out Instagram on our mobile phone and tag any new post with #ourhasthag to get it automatically pop-up on a e-paper picture frame.
Thanks to the technology behind V-Tablet, we just configure the device to point at the public URL of the new Node.js based service and presto – a nice picture frame, which will work for a couple of weeks before needing a recharge.
This article is a part of our technology showcase series, meant to inspire developers on what they could do with e-paper given proper tools like our Visionect V-Platform.
Tags